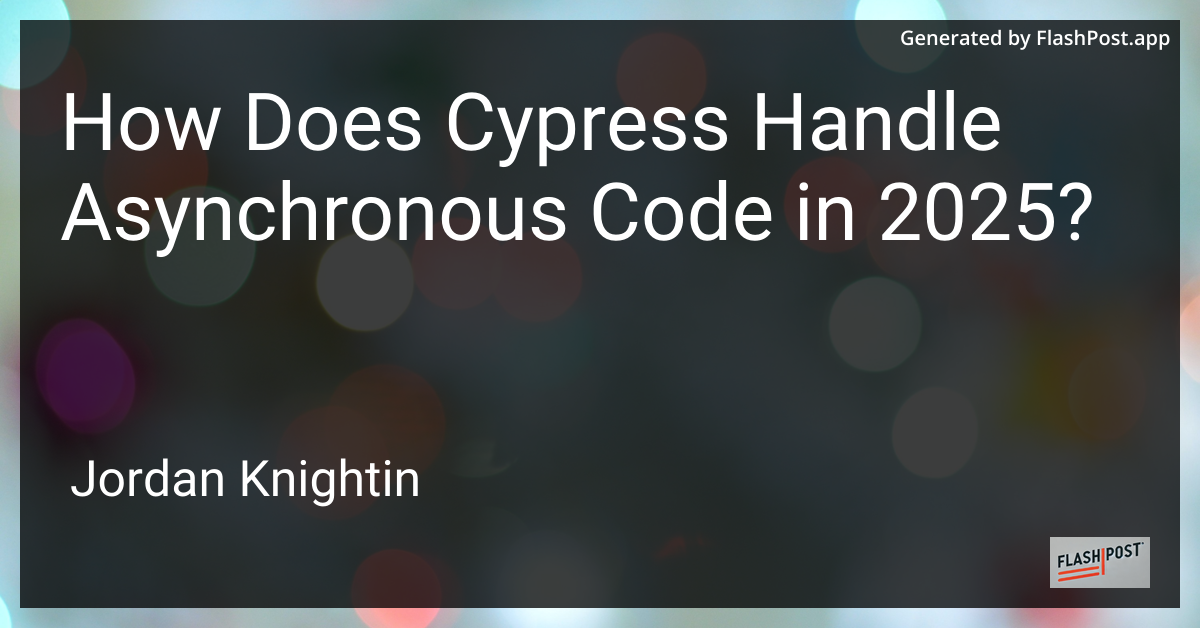
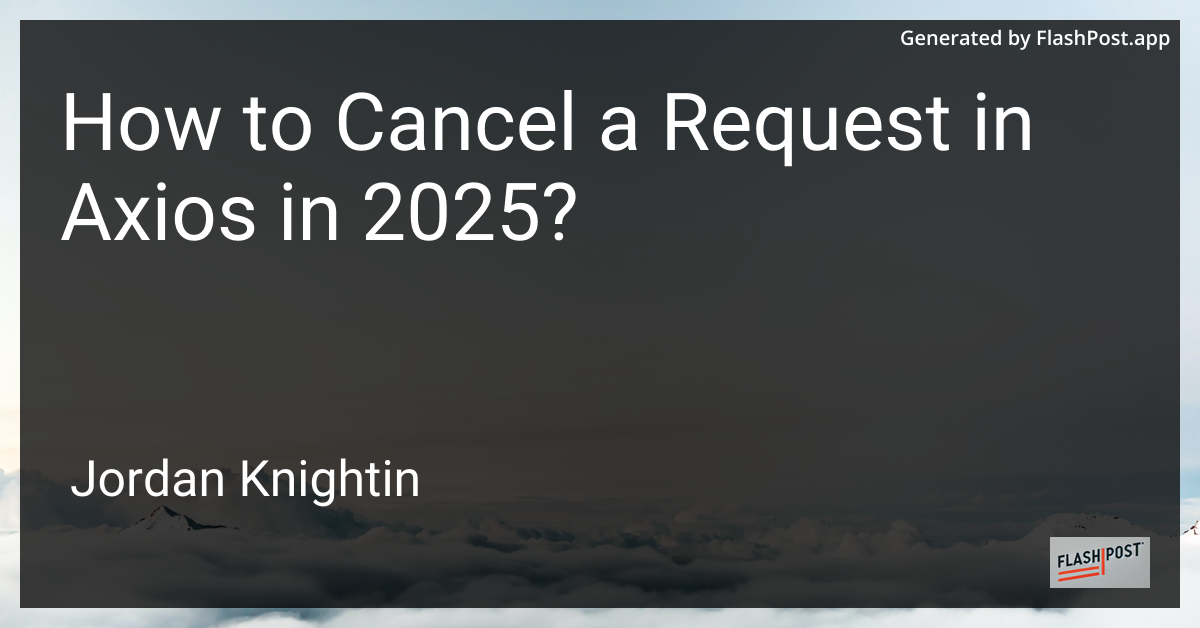
How to Cancel a Request in Axios in 2025?
In 2025, Axios remains one of the most popular HTTP clients for making asynchronous requests in JavaScript applications. Whether you’re working with modern JavaScript frameworks, like React or Vue, or integrating APIs, managing HTTP requests efficiently is crucial. One crucial aspect is the ability to cancel a request. Let’s explore how you can cancel a request in Axios seamlessly in 2025.
Understanding Request Cancellation
Cancelling requests in Axios has been a valuable feature for improving user experience and optimizing resource usage. Whether you are dealing with form submissions, API polling, or handling component lifecycle changes, there are occasions where cancelling ongoing requests is necessary.
Steps to Cancel a Request in Axios
Step 1: Install Axios
Firstly, if Axios isn’t already part of your project, you should install it using npm or yarn:
npm install axios
yarn add axios
Step 2: Use Axios CancelToken
In 2025, Axios provides the CancelToken
to control request cancellation. Here’s how you can implement it:
import axios from 'axios';
const source = axios.CancelToken.source();
axios.get('/user/12345', {
cancelToken: source.token
}).then((response) => {
console.log('User Data:', response.data);
}).catch((error) => {
if (axios.isCancel(error)) {
console.log('Request canceled:', error.message);
} else {
// handle error
}
});
// Cancel the request (the message parameter is optional)
source.cancel('Operation canceled by the user.');
Step 3: Use AbortController (Alternative Approach)
In compliance with the evolving Fetch API standards, Axios in 2025 also supports the AbortController
, which is a more modern way to handle cancellations:
const controller = new AbortController();
axios.get('/user/12345', {
signal: controller.signal
}).then((response) => {
console.log('User Data:', response.data);
}).catch((error) => {
if (error.name === 'CanceledError') {
console.log('Request canceled:', error.message);
} else {
// handle error
}
});
// To cancel the request
controller.abort();
Using the AbortController
aligns with native JavaScript standards and is expected to become the preferred method for request cancellation in libraries like Axios.
Conclusion
Cancelling requests in Axios is a crucial technique for efficient web application development in 2025. Whether it’s improving user interaction in JavaScript frameworks or managing asynchronous operations, proper request management enhances both performance and user experience.
For developers interested in testing their applications effectively, exploring a JavaScript testing framework is highly recommended. Additionally, understanding the differences and use cases of similar technologies is always advantageous, as outlined in the Java and JavaScript comparison for 2025.
Mastering techniques such as request cancellation ensures your applications are not only responsive but also resource-efficient, marking your skillset as highly relevant in the fast-evolving world of programming.