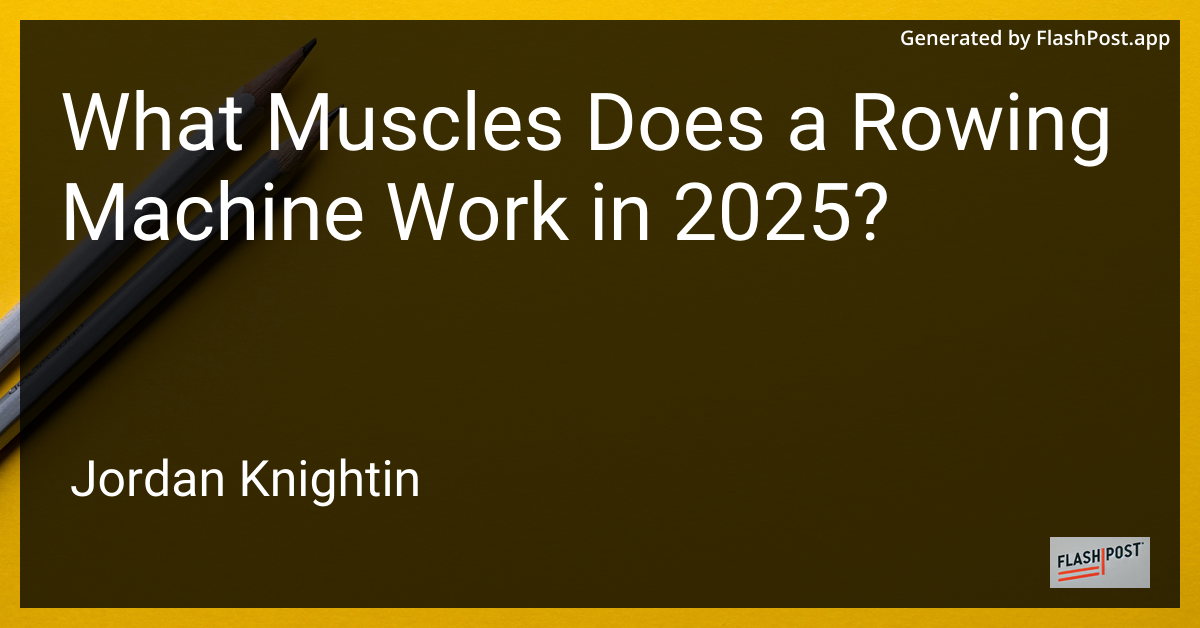
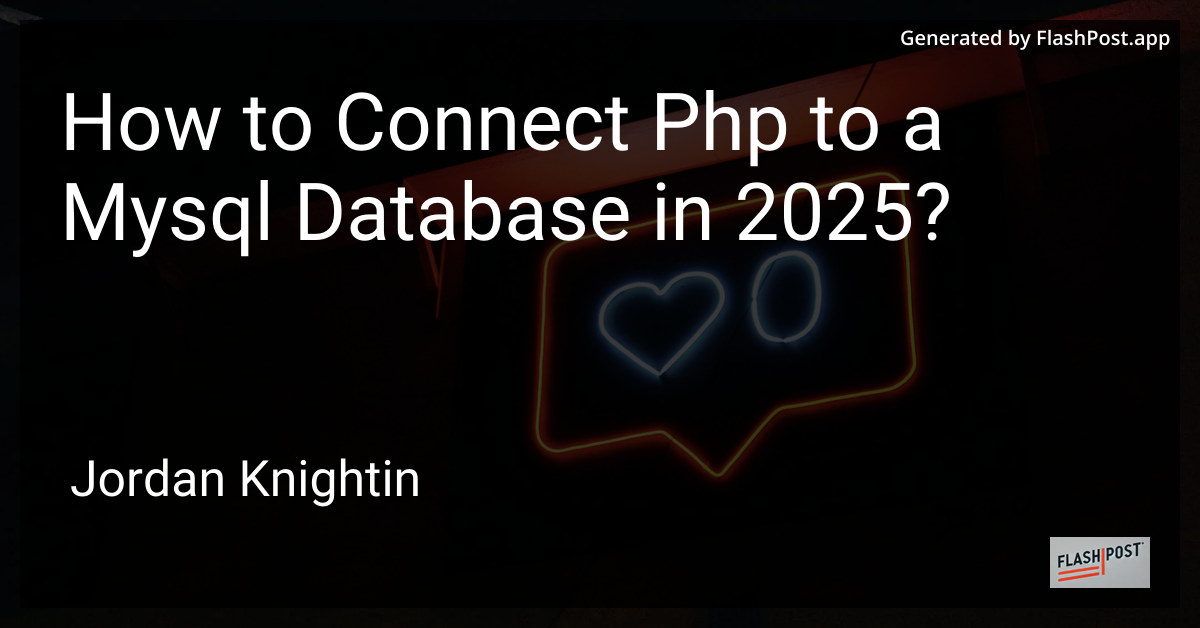
How to Connect Php to a Mysql Database in 2025?
Connecting PHP to a MySQL database is a fundamental task for web developers, enabling the creation of dynamic and interactive web applications. If you’re planning to establish a connection in 2025, this guide will provide you with the step-by-step process, ensuring your applications are robust and up-to-date with the latest standards.
Prerequisites
Before proceeding, ensure you have the following installed on your system:
- PHP 8.0 or later - Make sure your PHP version is up-to-date.
- MySQL 8.0 - A popular RDBMS for managing databases.
- Local Server Environment - Use XAMPP, WAMP, or a similar tool for local development.
Step 1: Start MySQL Service
First, ensure that the MySQL server is up and running on your local environment. You can refer to this comprehensive guide on starting MySQL service with XAMPP if you encounter any issues in doing so.
Step 2: Create a Database and User
You’ll need to create a database and a dedicated user to interact with the database.
CREATE DATABASE my_database;
CREATE USER 'my_user'@'localhost' IDENTIFIED BY 'secure_password';
GRANT ALL PRIVILEGES ON my_database.* TO 'my_user'@'localhost';
FLUSH PRIVILEGES;
Step 3: Connect PHP to MySQL
The next step involves writing PHP code to connect to your MySQL database. It’s advisable to use PDO (PHP Data Objects) for database connections, as it provides a robust and secure method that supports multiple database management systems.
Here’s a sample PHP script to connect via PDO:
<?php
$host = '127.0.0.1';
$db = 'my_database';
$user = 'my_user';
$pass = 'secure_password';
$charset = 'utf8mb4';
$dsn = "mysql:host=$host;dbname=$db;charset=$charset";
$options = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC,
PDO::ATTR_EMULATE_PREPARES => false,
];
try {
$pdo = new PDO($dsn, $user, $pass, $options);
echo "Connected successfully!";
} catch (\PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
Explanation:
- DSN (Data Source Name): It contains the host, database, and charset information.
- Options: PDO options that include error mode, fetch mode, and emulated prepares.
Step 4: Handling Connections
Proper connection handling is crucial for optimal performance. Be sure to close any open connections when they are no longer needed. For more details on how to do this, explore this insightful post on closing open MySQL connections.
Conclusion
By following these steps, you can effectively connect PHP to a MySQL database in 2025. For further learning or if you are interested in connecting other frameworks or languages to MySQL, check this interesting article about connecting Hibernate with MySQL.
Remember, always update your PHP and MySQL versions to benefit from the latest features and security patches. Happy coding!