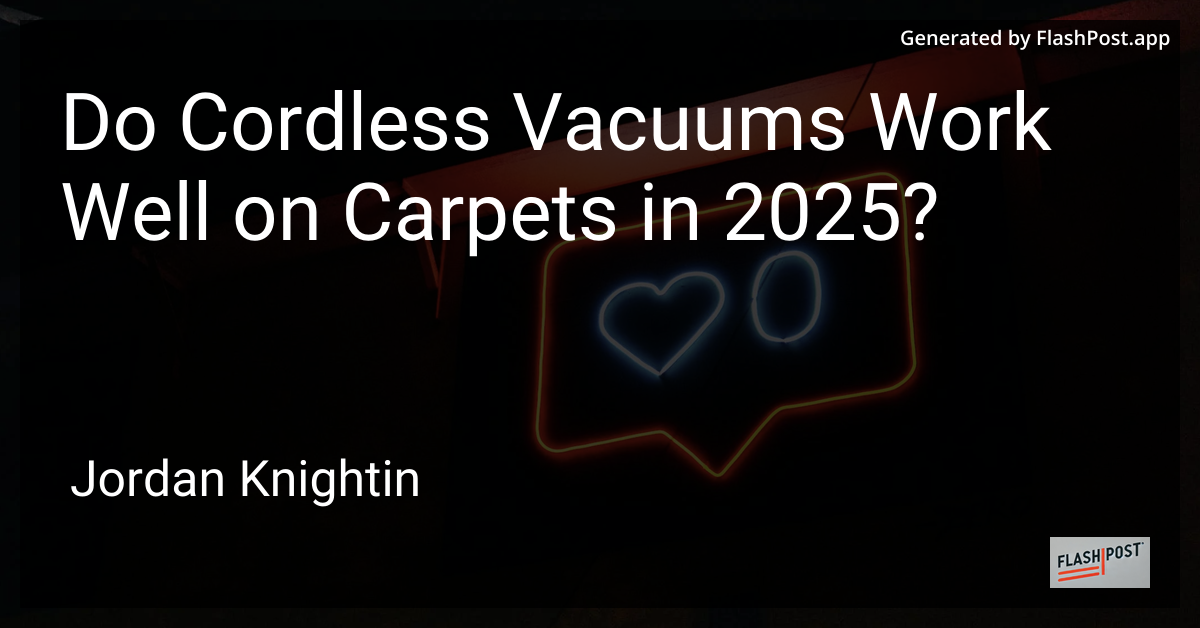
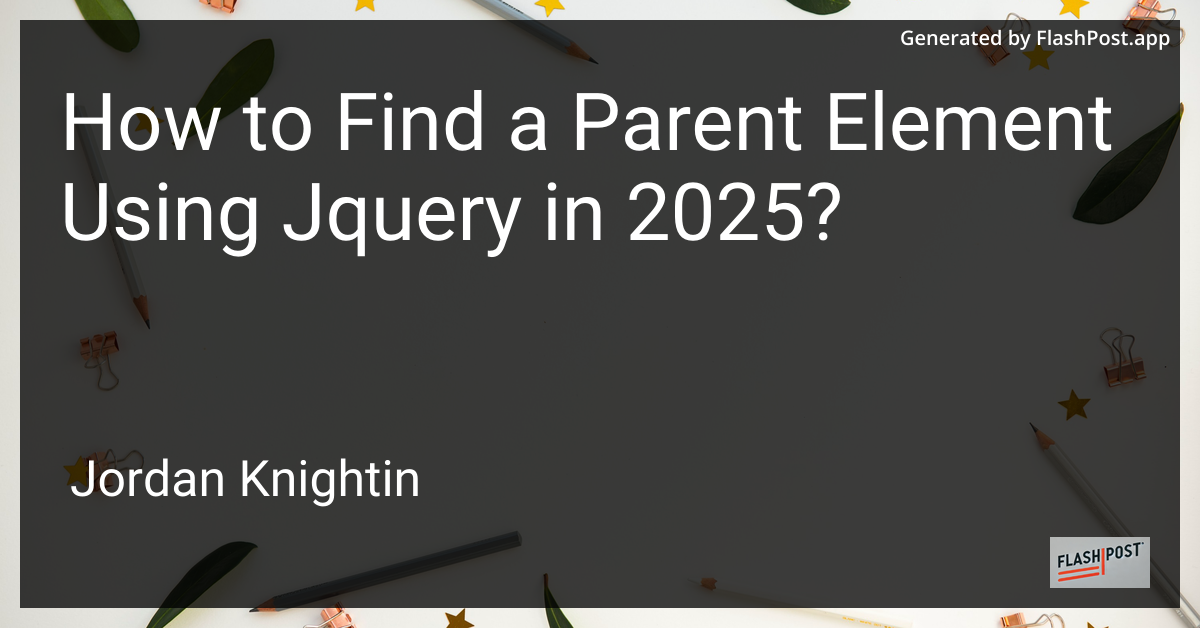
How to Find a Parent Element Using Jquery in 2025?
In the ever-evolving landscape of web development, jQuery continues to be a valuable tool for manipulating the DOM and handling events with ease. A common task for developers is finding a parent element of a specific element when navigating through the DOM. This process can be efficiently handled with jQuery by using the .parent()
and .parents()
methods. In this article, we will explore how to find a parent element using jQuery in 2025, and why this knowledge still holds relevance.
Why You Might Need to Find a Parent Element
Finding a parent element is important for several reasons:
- Event Handling: Sometimes, event handlers are attached to child elements, and you may need information from a parent.
- DOM Manipulation: You might want to manipulate not just a child element but also its parent to change styles, remove elements, or make structural changes.
- Form Validation: Validating and collecting data from form elements often requires traversing through parent nodes.
Using jQuery to Find Parent Elements
The .parent()
Method
The .parent()
method is used to get the immediate parent of each element in the set of matched elements. Here is a basic usage example:
$(document).ready(function() {
// Selecting the immediate parent of a specific element
$('#childElement').parent().css('background-color', '#f0f0f0');
});
In this example, the parent of the element with the ID childElement
will have its background color changed. The .parent()
method is ideal when you need to interact directly with an element’s immediate parent.
The .parents()
Method
For situations where you need to traverse multiple levels up the DOM tree, the .parents()
method comes into play. This method returns all ancestor elements, up to but not including the root element. You can also provide an optional selector to filter the results:
$(document).ready(function() {
// Finding all ancestor elements of the specified element
$('#childElement').parents().css('border', '1px solid #000');
// Find specific parent with a class
$('#childElement').parents('.specificParentClass').css('border', '2px dashed #ff0000');
});
The .parents()
method is particularly useful when dealing with nested structures, allowing developers to target specific nodes higher up in the hierarchy based on conditions.
Best Practices for Using jQuery in 2025
- Performance Considerations: While jQuery offers robust methods to manipulate the DOM, it’s essential to consider performance impacts, especially in applications with large DOM trees.
- Modern JavaScript: With advancements in JavaScript, such as ES6, it’s worth sometimes combining jQuery with vanilla JavaScript for optimized performance and simplicity.
- Maintainability: Keep your code clean and well-documented, especially when traversing complex DOM hierarchies. This ensures that your code remains maintainable and scalable.
Further Reading
- jQuery AJAX: Preventing Caching Issues - Discover how to manage and prevent caching when using jQuery AJAX.
- Integrating jQuery with Yii2 - Learn how to seamlessly add jQuery to Yii2 applications.
- Styling iFrame Content using jQuery - Explore techniques for changing and adding CSS inside of an iFrame using jQuery.
Conclusion
Even as web technologies evolve, jQuery remains a powerful library for DOM manipulation and event handling. Understanding how to efficiently find a parent element using methods like .parent()
and .parents()
continues to be a valuable skill for developers. By keeping current with best practices and integrating knowledge with other technologies, developers can continue to leverage jQuery effectively in their web projects throughout 2025 and beyond.