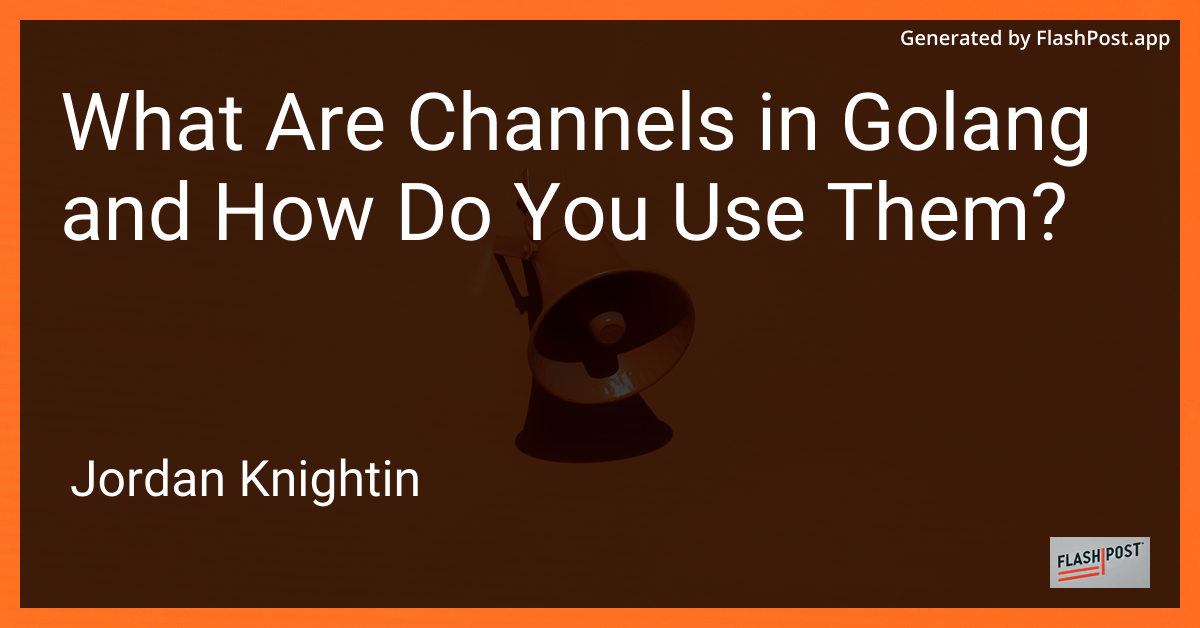
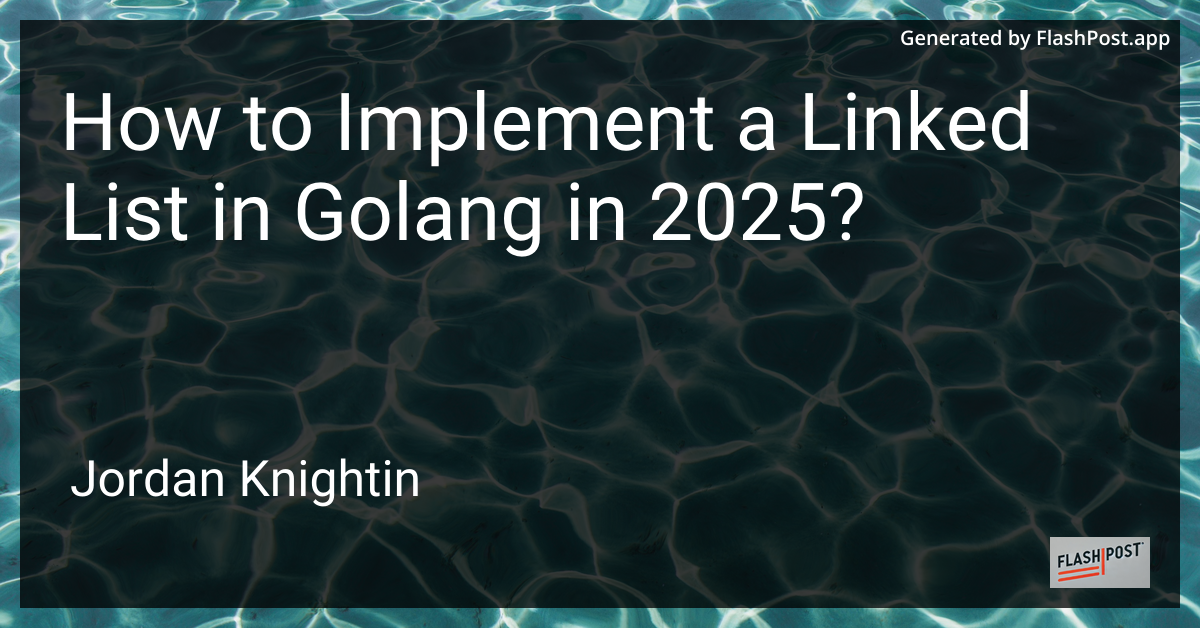
How to Implement a Linked List in Golang in 2025?
title: How to Implement a Linked List in GoLang in 2025 description: A comprehensive guide to implementing a linked list in GoLang in 2025. Optimize your GoLang development skills with this step-by-step tutorial. keywords: golang, linked list, data structures, go programming, 2025 author: Your Name published: 2025-01-15
In the realm of programming, understanding data structures is crucial for crafting efficient and effective software solutions. One fundamental data structure is the linked list. In this article, you will learn how to implement a linked list in GoLang, a powerful language known for its efficiency and robustness, in the year 2025.
What is a Linked List?
A linked list is a linear data structure where each element (called a node) contains a reference (or link) to the next node in the sequence. This structure allows for efficient insertions and deletions, which can be more challenging with arrays.
Why Use Linked Lists in GoLang?
GoLang, or Go, is a language favored for its simplicity and performance. Using linked lists in GoLang can enhance performance in scenarios where dynamic data storage is necessary. As of 2025, GoLang remains a top choice for systems programming due to its ability to handle concurrent operations efficiently.
Implementing a Linked List in GoLang
Below is a step-by-step process to implement a linked list in GoLang.
Step 1: Define the Node Structure
First, you need to create a Node
struct. Each node will hold data and a pointer to the next node.
package main
import "fmt"
// Node represents a node in the linked list
type Node struct {
data int
next *Node
}
Step 2: Create the LinkedList Structure
Next, define a LinkedList
struct to manage the operations on the linked list.
// LinkedList represents the linked list
type LinkedList struct {
head *Node
}
Step 3: Initialize a New Linked List
You’ll now create a method to initialize a new linked list.
// Initialize a new empty linked list
func (list *LinkedList) Init() {
list.head = nil
}
Step 4: Implement Insertion Method
Let’s implement a method to insert new nodes into the linked list.
// Insert adds a new node with the data to the linked list
func (list *LinkedList) Insert(data int) {
newNode := &Node{data: data}
if list.head == nil {
list.head = newNode
} else {
current := list.head
for current.next != nil {
current = current.next
}
current.next = newNode
}
}
Step 5: Implement Deletion Method
Create a method to delete a specified node from the linked list.
// Delete removes a node with the specified data
func (list *LinkedList) Delete(data int) {
if list.head == nil {
return
}
if list.head.data == data {
list.head = list.head.next
return
}
current := list.head
for current.next != nil && current.next.data != data {
current = current.next
}
if current.next != nil {
current.next = current.next.next
}
}
Step 6: Display the Linked List
Finally, implement a method to display the linked list.
// Display prints all nodes in the linked list
func (list *LinkedList) Display() {
current := list.head
for current != nil {
fmt.Printf("%d -> ", current.data)
current = current.next
}
fmt.Println("nil")
}
Complete Example
Here is how you can use the linked list:
func main() {
list := &LinkedList{}
list.Init()
list.Insert(10)
list.Insert(20)
list.Insert(30)
fmt.Println("Linked List after insertion:")
list.Display()
list.Delete(20)
fmt.Println("Linked List after deletion of 20:")
list.Display()
}
Conclusion
Implementing a linked list in GoLang is straightforward and enhances your ability to manage dynamic datasets efficiently. As technology progresses, mastering such data structures in GoLang ensures you’re prepared to tackle complex problems in the modern programming landscape of 2025.
Additional Resources
- Curious about the salary of a Golang programmer in China in 2025? Find out more here.
- Enhance your Go skills with a detailed tutorial on using context in GoLang in 2025. Check it out here.
- Want to learn about XML parsing in GoLang? Explore the tutorial here.
By following these steps and utilizing the additional resources, you’re on your way to becoming proficient in GoLang and implementing effective data structures for your projects in 2025.