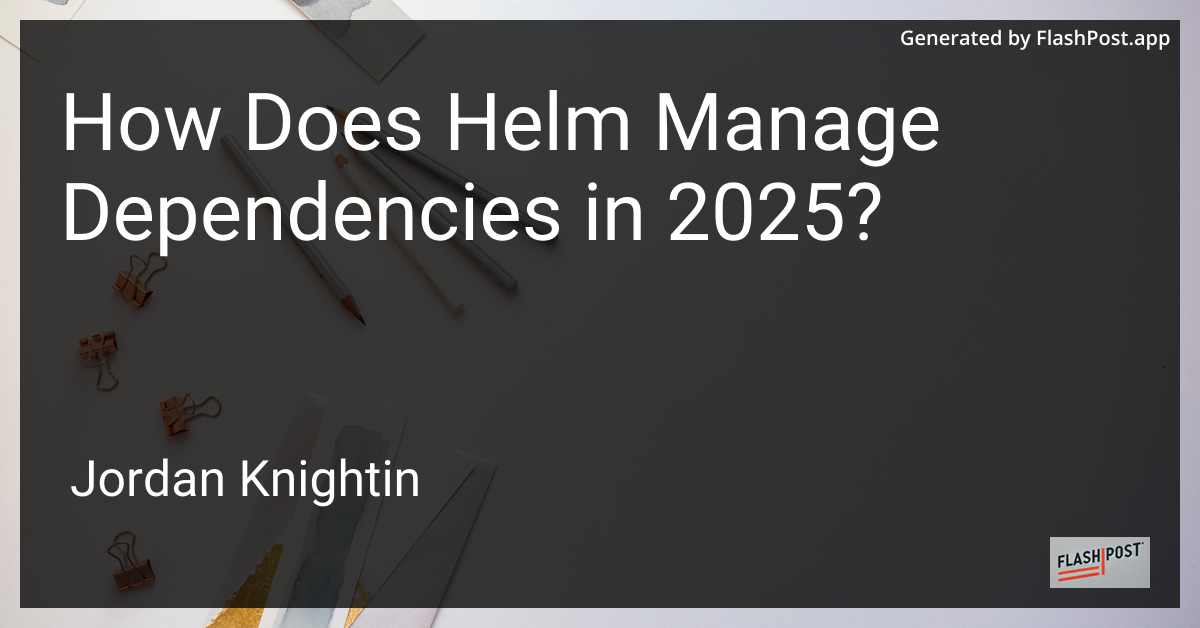
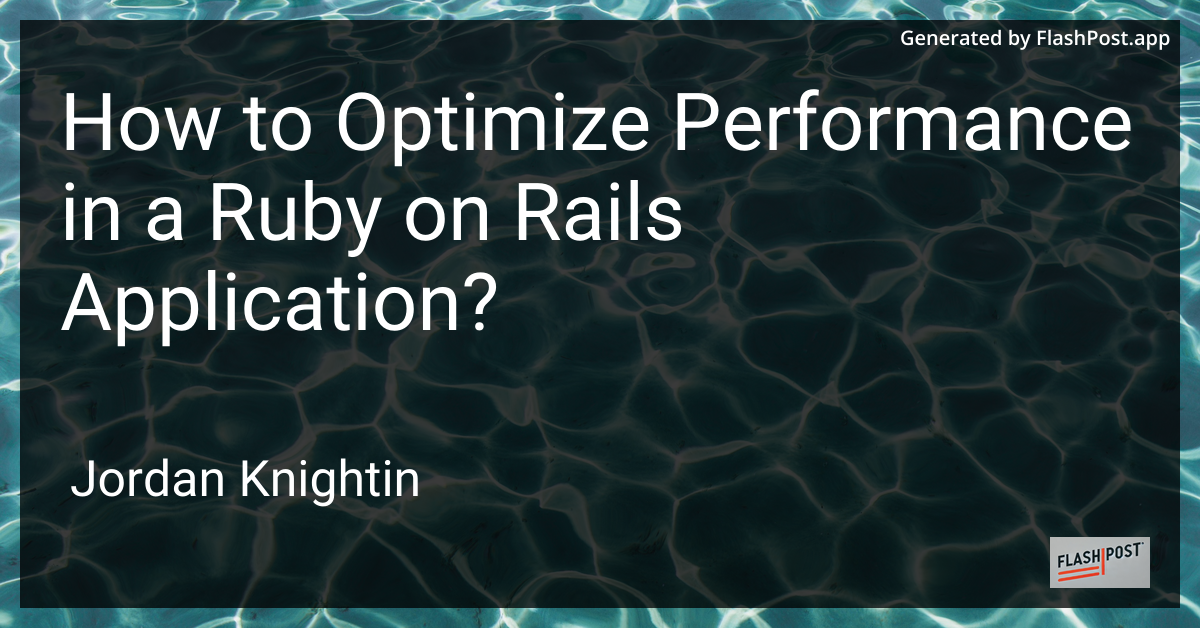
How to Optimize Performance in a Ruby on Rails Application?
Ruby on Rails is a powerful framework for building web applications, known for its ease of use and flexibility. However, as applications grow, performance can become an issue. Optimizing a Ruby on Rails application is crucial to ensure it runs smoothly and efficiently, providing users with a seamless experience. In this article, we will explore key strategies for optimizing the performance of your Ruby on Rails application.
1. Utilize Efficient Data Fetching
Data fetching can greatly impact performance, especially when dealing with large datasets. To enhance performance, you should:
- Use Eager Loading: Avoid N+1 query problems by using
includes
to load associated records simultaneously. - Select Required Fields Only: Instead of fetching all fields, use
select
to retrieve only the necessary columns. - Optimize SQL Queries: Regularly review and refactor SQL queries to ensure they are optimized for performance.
For more details on fetching data effectively, check out this article on data fetching in Ruby on Rails.
2. Implement Caching
Caching is one of the most effective ways to boost performance:
- Page Caching: Cache entire pages to reduce server load.
- Fragment Caching: Cache parts of a page, such as sidebar widgets.
- Russian Doll Caching: A nested caching approach that invalidates only specific parts of a cache.
Rails comes with built-in support for caching. Configure your application to use cache stores like Memcached or Redis for better performance.
3. Optimize Asset Pipeline
The asset pipeline in Rails manages JavaScript, CSS, and images, and optimizing it can reduce load times:
- Minify and Concatenate: Minify CSS and JavaScript files and concatenate them into single files to reduce HTTP requests.
- Use Content Delivery Network (CDN): Serve assets over a CDN to reduce latency and load times.
- Precompile Assets: Precompile assets in production for faster serving.
4. Database Indexing
Indexing your database tables helps speed up query performance:
- Identify Frequently Queried Fields: Index fields that are regularly queried.
- Use Compound Indexes: For queries involving multiple columns, create compound indexes to improve search efficiency.
5. Background Job Processing
Long-running tasks can slow down your application if run synchronously:
- Use Background Processing: Tools like Sidekiq, Resque, or Delayed Job can offload tasks to a background queue, allowing the main application to continue processing requests without delay.
6. Rails Environment Configuration
Ensure that your Rails environment is configured for performance:
- Production Mode: Run your application in production mode to enable performance optimizations.
- Optimize Logging: Reduce log level and avoid logging unnecessary information in production.
7. Monitor and Analyze Performance
Regularly monitor your application’s performance using tools like:
- New Relic: Provides insights into application performance and bottlenecks.
- ScoutAPM: Offers detailed performance monitoring for Rails applications.
Conclusion
Optimizing a Ruby on Rails application for performance is essential as your user base grows. Implementing efficient data fetching, leveraging caching mechanisms, optimizing the asset pipeline, indexing databases, handling background jobs, configuring the environment correctly, and using monitoring tools can significantly enhance performance. For further reading on Ruby on Rails development and building complex systems, check out this expert guide on Ruby on Rails development and explore how to build a forum website with Ruby on Rails.
By following these best practices, you can ensure your Ruby on Rails application remains fast, responsive, and scalable.