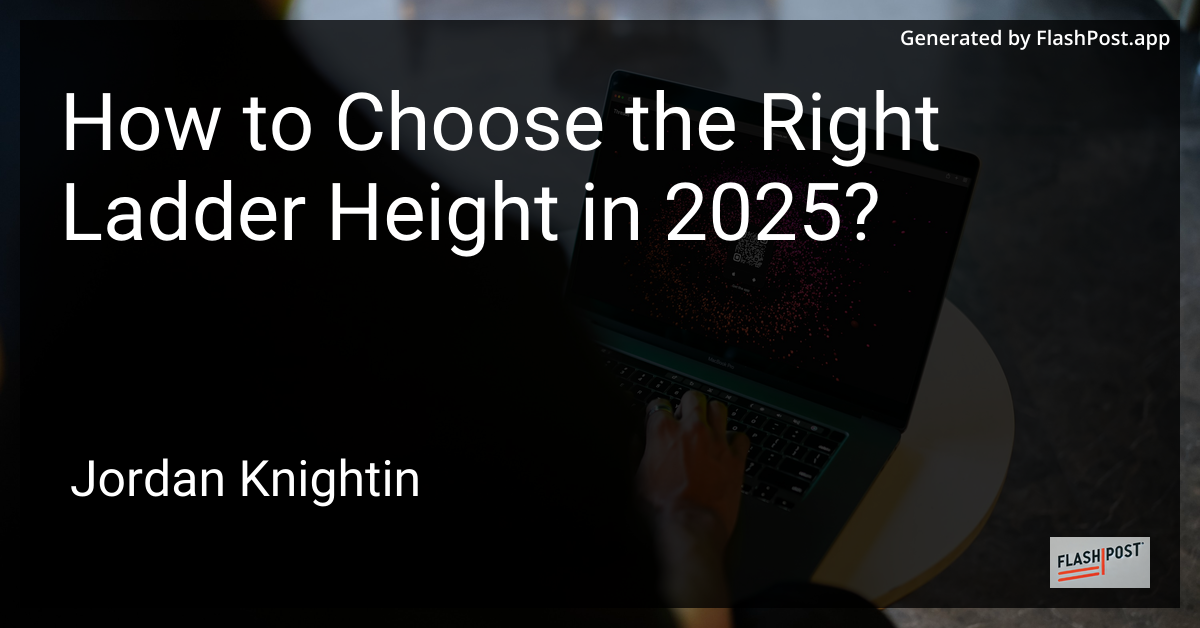
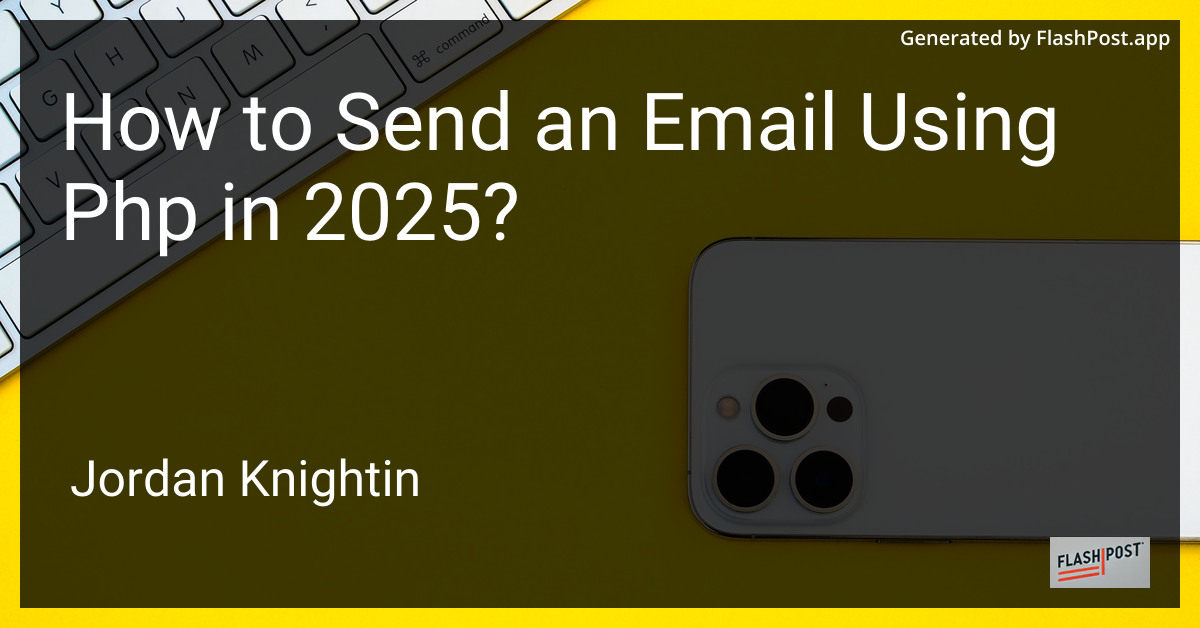
How to Send an Email Using Php in 2025?
title: How to Send an Email Using PHP in 2025 description: A comprehensive guide on sending emails using PHP in 2025. Learn the best practices, libraries, and tools for effective email functionality. keywords: PHP, email, send mail, PHPMailer, SMTP, 2025 author: Your Name date: 2025-03-20
Sending emails programmatically has always been an essential feature in web development. If you’re a PHP developer looking to incorporate email functionalities in 2025, you’re in the right place. In this guide, we’ll explore how to send an email using PHP, including the latest practices, libraries, and considerations for ensuring deliverability and security.
Prerequisites
Before we dive in, ensure you have the following:
- Access to a PHP development environment: You can set this up on your local machine or use a cloud-based solution.
- An SMTP server: This is essential for sending emails. You can use services like Gmail, SendGrid, or Mailgun.
- PHPMailer library: One of the most popular libraries for sending emails using PHP.
Step-by-Step Guide
1. Install PHP and Composer
Ensure that you have PHP installed on your machine. Composer, the PHP dependency manager, is also required. If you’re unfamiliar with Composer, check out this detailed guide on PHP dependency management.
2. Install PHPMailer
PHPMailer is known for its ease of use and powerful features. Use Composer to install it:
composer require phpmailer/phpmailer
3. Configure PHPMailer
Here’s a basic configuration to send an email using PHPMailer’s SMTP:
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
//Server settings
$mail->SMTPDebug = 0; // Enable verbose debug output
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.example.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = 'user@example.com'; // SMTP username
$mail->Password = 'password'; // SMTP password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('from@example.com', 'Mailer');
$mail->addAddress('joe@example.net', 'Joe User'); // Add a recipient
$mail->addReplyTo('info@example.com', 'Information');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
?>
4. Handle Errors and Debugging
Error handling and debugging are crucial, especially when working with email functionalities. It’s advisable to implement detailed logging and error-catching mechanisms. Learn more about modern PHP debugging techniques.
5. Testing Your Email Functionality
Before deploying your email-sending functionality, thorough testing is necessary. Utilize PHP testing frameworks like PHPUnit. This guide on how to run specific tests in PHPUnit in 2025 will provide insights into efficient testing methodologies.
Best Practices for 2025
- Ensure Email Deliverability: Use SPF, DKIM, and DMARC to prevent your emails from being marked as spam.
- Maintain Security: Regularly update libraries and use secure connections for SMTP.
- Monitor Performance: Track email bounces, open rates, and other analytics.
Conclusion
Sending an email using PHP in 2025 is streamlined with robust libraries like PHPMailer and modern tools like Composer for dependency management. By adhering to best practices and thoroughly testing your application, you can ensure reliable and secure email functionality in your projects. Keep abreast of the latest advancements and continuously improve your implementation techniques for optimal results.