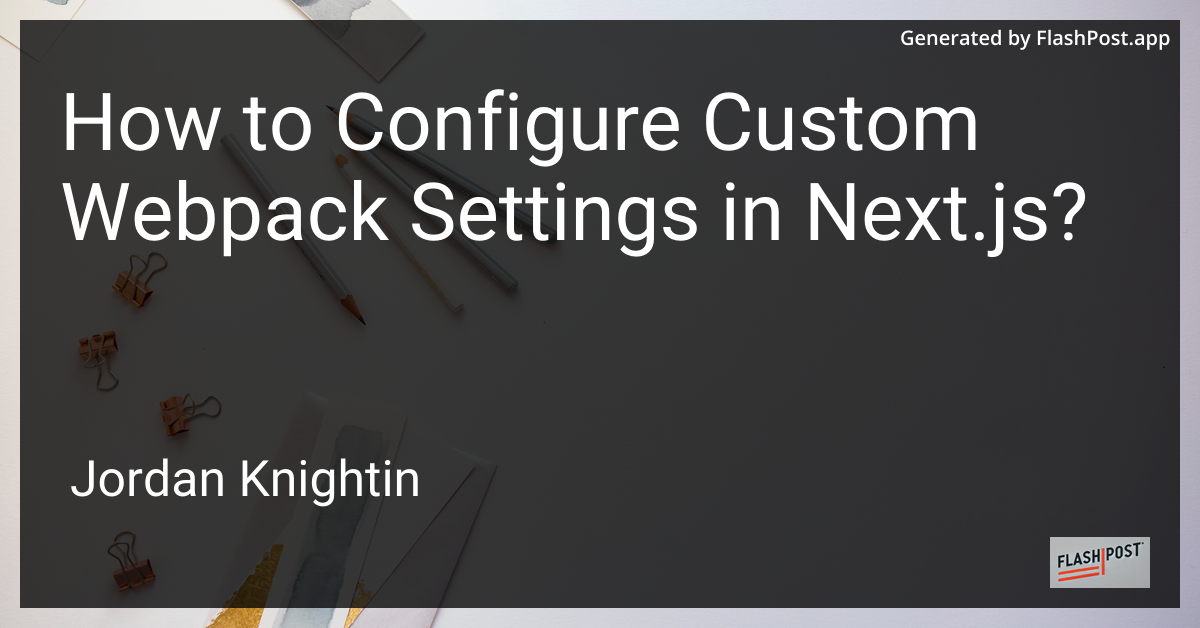
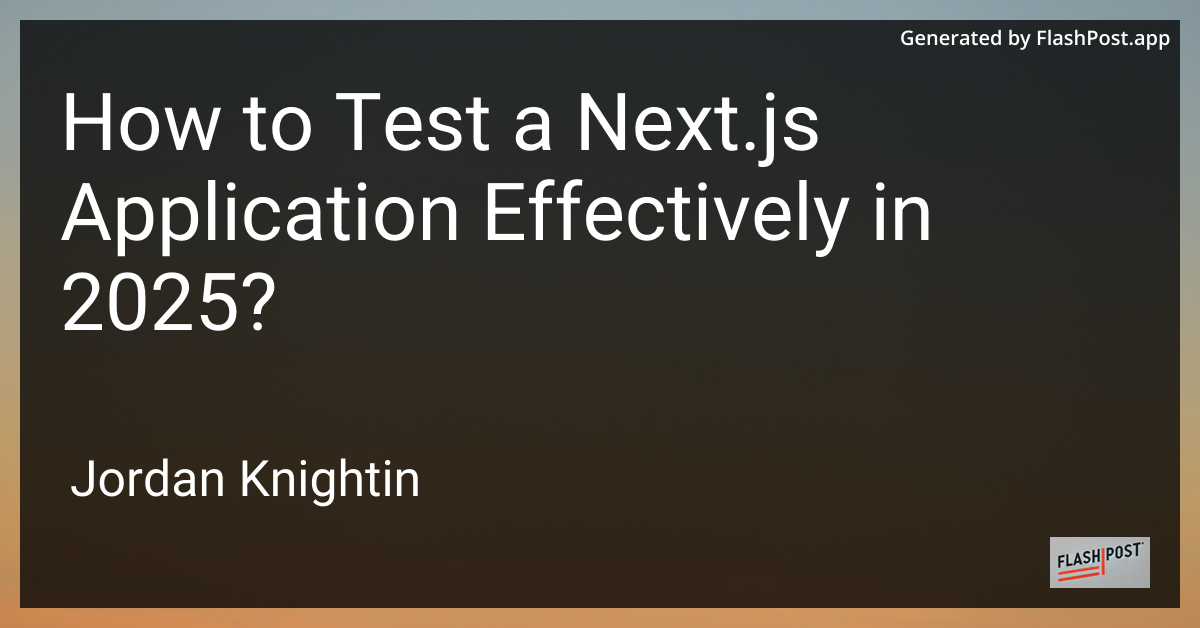
How to Test a Next.js Application Effectively in 2025?
Testing is a crucial part of the development process, especially in high-performance frameworks like Next.js. As we move into 2025, the methods and tools for testing a Next.js application have evolved, ensuring applications are robust, efficient, and bug-free. This article will explore the best practices and tools to test a Next.js application effectively.
Understanding Testing Types
Before diving into tools and strategies, it’s essential to understand the different types of testing you can perform on a Next.js application:
- Unit Testing: Focuses on testing individual components or functions.
- Integration Testing: Ensures that combined parts of the application function together as expected.
- End-to-End (E2E) Testing: Tests the complete flow of your application from the user’s perspective.
- Performance Testing: Evaluates how your application performs under load.
Best Practices for Testing Next.js Applications
1. Utilize Jest for Unit Testing
Jest, a delightful JavaScript testing framework, works perfectly with Next.js for unit tests. It’s efficient and widely used in the Next.js community.
-
Setup Jest with Next.js:
npx create-next-app@latest my-next-app cd my-next-app npm install --save-dev jest @testing-library/react @testing-library/jest-dom
-
Create a Test Script: Update your
package.json
to include a test script."scripts": { "test": "jest" }
2. Integration Testing with Testing Library
The Testing Library suite, with its focus on user-centric testing, is an excellent choice for integration testing.
-
Installation:
npm install --save-dev @testing-library/react
-
Example Test Case:
import { render, screen } from '@testing-library/react'; import Home from '../pages/index'; test('renders home page', () => { render(<Home />); const linkElement = screen.getByText(/welcome to next\.js/i); expect(linkElement).toBeInTheDocument(); });
3. End-to-End Testing with Cypress
Cypress is a powerful E2E testing tool that simulates real user interactions in your application.
-
Setting Up Cypress:
npm install --save-dev cypress
-
Writing Your First E2E Test: Cypress tests are straightforward and user-focused, ideal for testing real-world scenarios.
4. Performance Testing with Lighthouse
Lighthouse, an open-source tool by Google, is perfect for analyzing and improving your app’s performance metrics. You can integrate Lighthouse audits in your CI/CD pipeline to catch performance regressions early.
Advanced Testing Strategies
Mocking External Services
Use tools like MSW (Mock Service Worker) to mock external API calls during testing, allowing you to test various scenarios without relying on the actual external services.
Snapshot Testing
Incorporate snapshot testing to track UI changes. Jest can help you manage snapshots efficiently, aiding in visual regression testing.
Additional Resources
- To optimize images, an crucial aspect of Next.js applications, read this guide on Next.js image SEO.
- Implementing server-side rendering (SSR) correctly is crucial for SEO and performance. Check out this resource on Next.js SSR Implementation.
- For managing different environments, learn how to set the base URL by referring to Next.js Base URL.
Conclusion
Testing a Next.js application effectively in 2025 requires a combination of the right tools and strategies. By leveraging Jest, Testing Library, Cypress, and Lighthouse, you can ensure your application is well-tested and performs optimally. Always keep your testing environment updated with the best practices and tools to maintain your edge in efficient application development.
Happy testing!