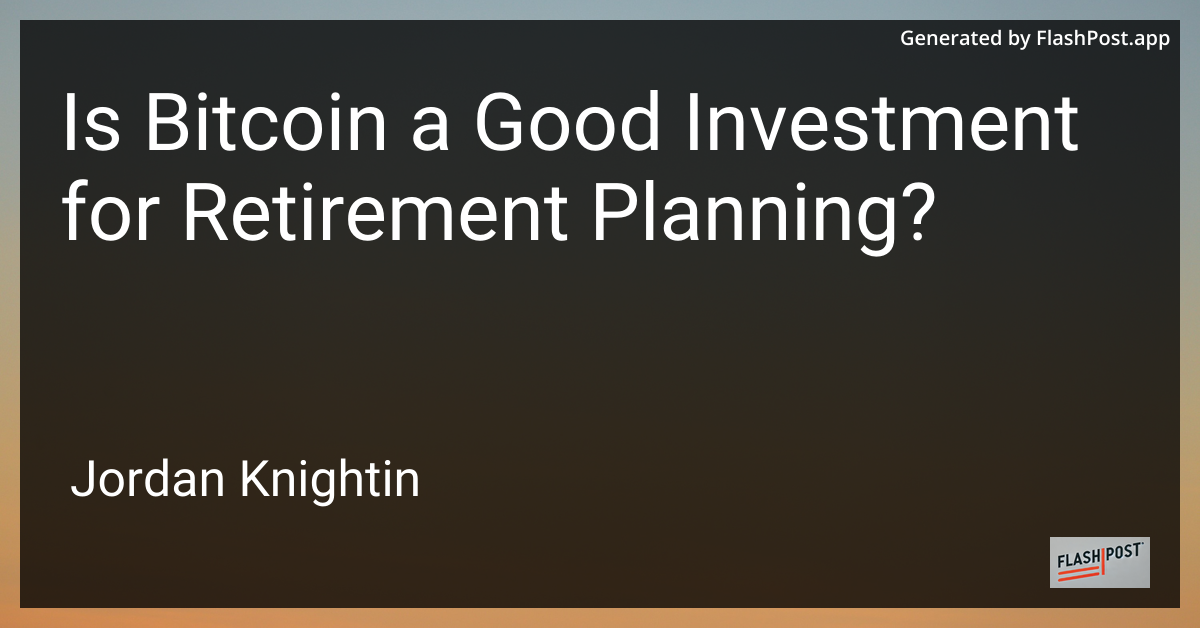
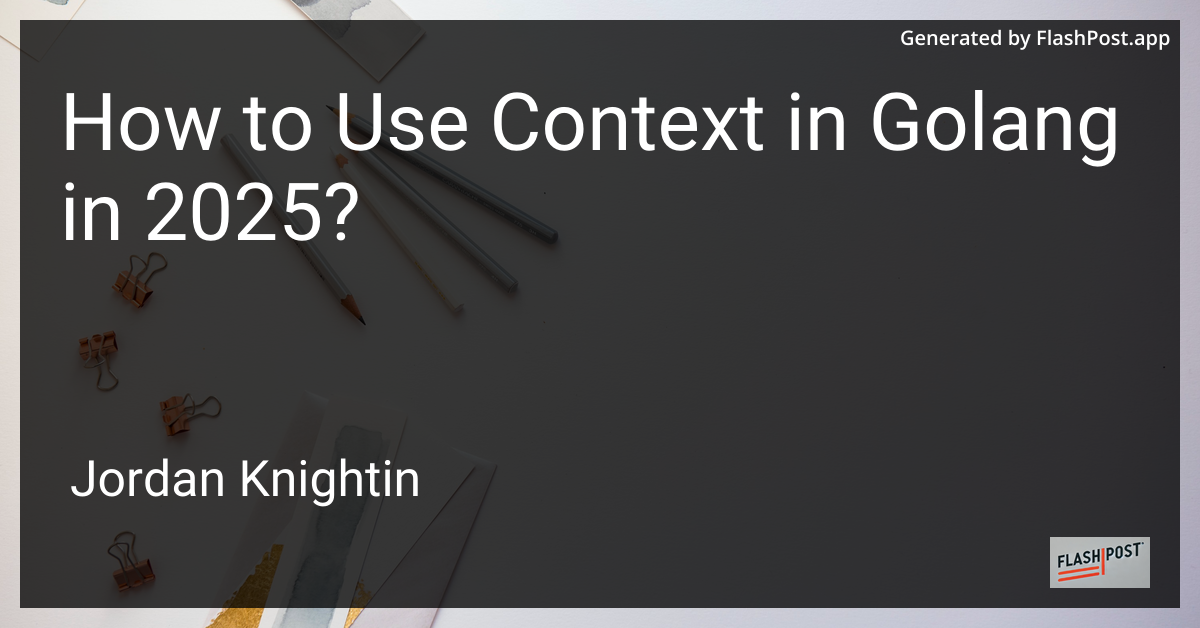
How to Use Context in Golang in 2025?
The Go programming language, often referred to as Golang, continues to evolve with every passing year. As we head into 2025, mastering the use of context in Golang remains crucial for developing robust and efficient applications. The context
package in Go plays a vital role in handling deadlines, cancellations, and request-scoped data, making it indispensable for modern software development.
Understanding Context in Golang
The context
package in Go is designed to simplify the management of deadlines, cancellations, and the transmission of request-scoped data across API boundaries and between processes. Here’s how you can effectively use context in your Go projects:
Key Features of Context
-
Cancellation Signals: Contexts allow you to propagate cancellation signals, enabling you to terminate operations early to save computing resources.
-
Deadlines and Timeouts: You can set a deadline or timeout for an operation, ensuring that it doesn’t run indefinitely.
-
Request-Scoped Data: Contexts help in passing request-scoped values across function calls, making your application more modular and cleaner.
Creating and Using Context
To illustrate how to implement context, consider the following code example:
package main
import (
"context"
"fmt"
"time"
)
func main() {
// Create a context with a timeout of 5 seconds
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
// Simulate a long-running operation
done := make(chan struct{})
go func() {
time.Sleep(3 * time.Second) // Simulate work
fmt.Println("Operation completed")
close(done)
}()
select {
case <-done:
fmt.Println("Work successfully completed within the deadline.")
case <-ctx.Done():
fmt.Println("Operation cancelled:", ctx.Err())
}
}
Best Practices for Using Context
- Pass Context Explicitly: Always pass the context as the first parameter to functions that need it.
- Avoid Storing Contexts in Structs: Pass context explicitly rather than storing it across multiple function calls.
- Handle Errors Gracefully: Use
ctx.Err()
to check for cancellation or timeout errors.
Enhancing Your Golang Skills
To become proficient in using context and other advanced features in Golang, you may find the following resources useful:
- Learn how to retrieve system information in Golang to enhance your Golang applications with system-level insights.
- Discover techniques for passing complex data structures by exploring how to pass a map parameter in Golang.
- Master data presentation by learning about template usage in Golang.
By following these guidelines and expanding your skills with the above resources, you’ll be well-equipped to harness the full potential of context in Golang as you develop future-ready applications in 2025 and beyond.
Conclusion
In 2025, utilizing the context package in Golang remains a cornerstone for effective application development. By leveraging context for cancellation signals, deadlines, and scoped data management, you can enhance the performance and reliability of your applications. Keep honing your skills and stay updated with the latest developments in the Go ecosystem to remain at the forefront of software innovation.