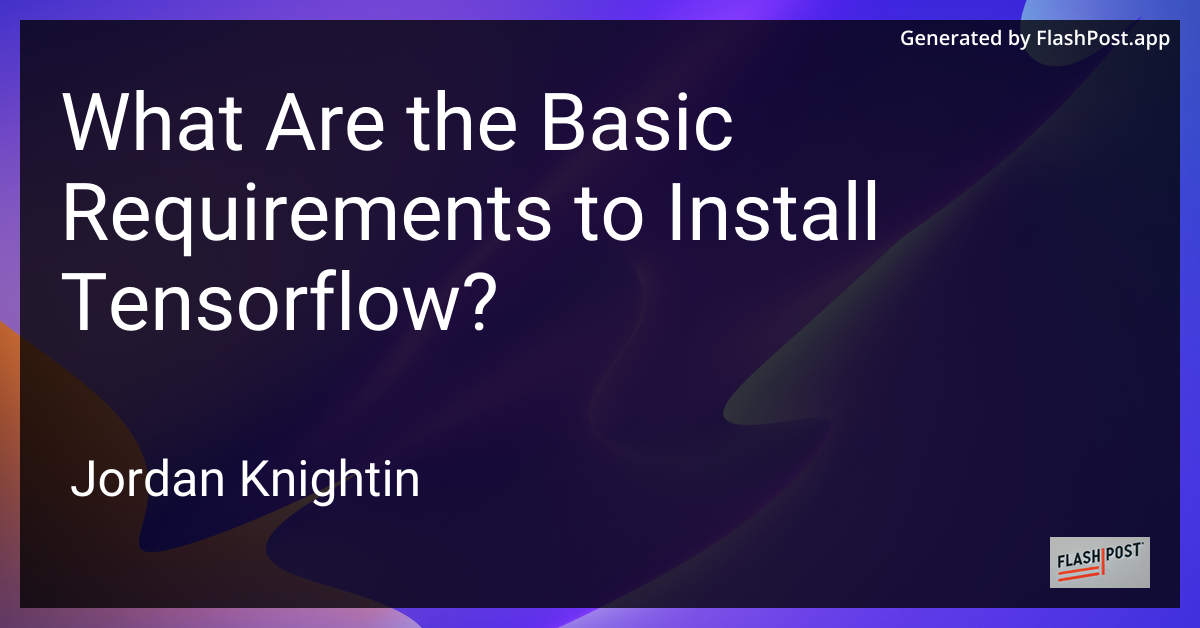
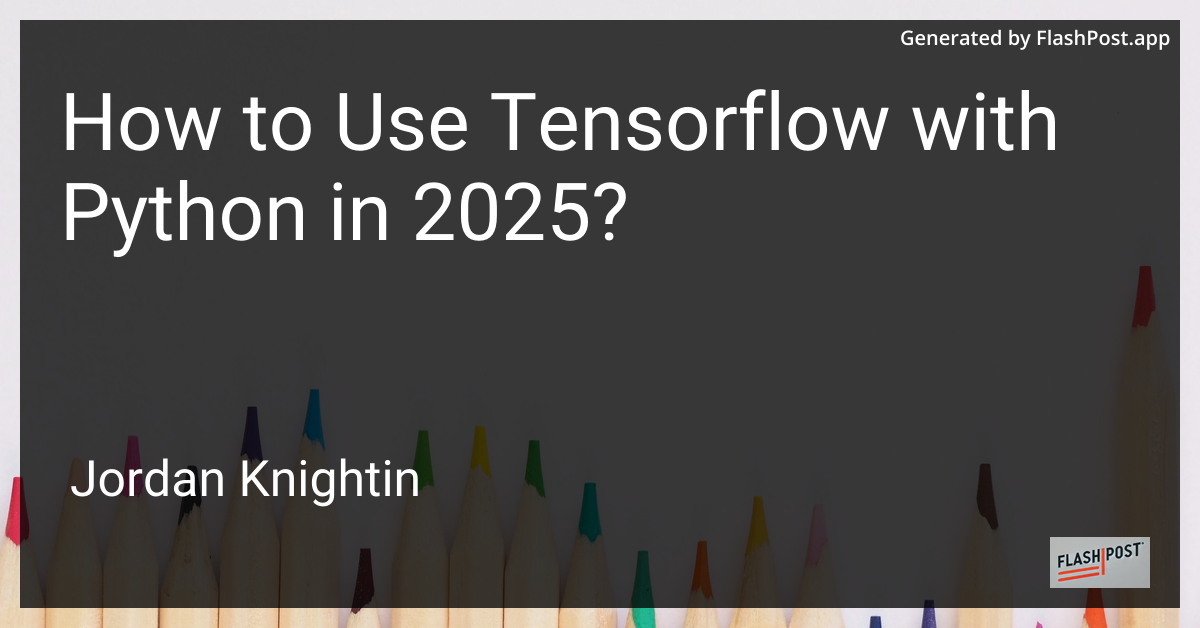
How to Use Tensorflow with Python in 2025?
In 2025, TensorFlow continues to be one of the most powerful tools for machine learning and deep learning. Whether you’re new to data science or an experienced practitioner, harnessing the power of TensorFlow with Python can catalyze amazing results in AI applications. This guide will equip you with the knowledge to effectively utilize TensorFlow with Python, providing you with the foundational skills necessary for tackling complex machine learning tasks.
Getting Started with TensorFlow
Installation
To start using TensorFlow, you first need to install it. Here’s how you can install TensorFlow in Python:
pip install tensorflow
Make sure you have Python 3.8 or above installed on your system, as TensorFlow in 2025 supports only newer versions due to enhanced features and security updates.
Setting Up Your Environment
It is highly recommended to use virtual environments to manage your project dependencies effectively. You can create a virtual environment using venv
:
python3 -m venv my_tensorflow_env
source my_tensorflow_env/bin/activate # On Windows use: my_tensorflow_env\Scripts\activate
Once your environment is activated, reinstall TensorFlow within this environment.
Building Your First Model
Let’s dive into building a simple neural network using TensorFlow. We will create a basic model for classifying images from the Fashion MNIST dataset.
Import Libraries
import tensorflow as tf
from tensorflow.keras import layers, models
import numpy as np
Load and Prepare Data
fashion_mnist = tf.keras.datasets.fashion_mnist
(train_images, train_labels), (test_images, test_labels) = fashion_mnist.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
Build the Model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)),
layers.Dense(128, activation='relu'),
layers.Dense(10)
])
Compile the Model
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
Train the Model
model.fit(train_images, train_labels, epochs=10)
Evaluate the Model
Make sure to evaluate your TensorFlow model’s accuracy to understand its performance:
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print(f'\nTest accuracy: {test_acc}')
Advanced Tips for TensorFlow in 2025
Efficiently Running TensorFlow on NVIDIA GPUs
Leverage the power of GPUs to speed up your model training process. Check out this guide on running TensorFlow efficiently on NVIDIA GPUs.
Manipulating Model Neurons
TensorFlow allows you to fine-tune your models even further. Learn how to remove a specific neuron inside your model for custom optimizations.
Conclusion
TensorFlow’s compatibility with Python makes it an exceptional choice for AI projects in 2025. Whether you’re training models or deploying them in production, the synergy between TensorFlow and Python ensures robust and efficient execution of your data-driven applications. Harness these insights and elevate your AI competencies to new heights today.
Feel free to explore the advanced resources linked above to further deepen your understanding and capabilities with TensorFlow.