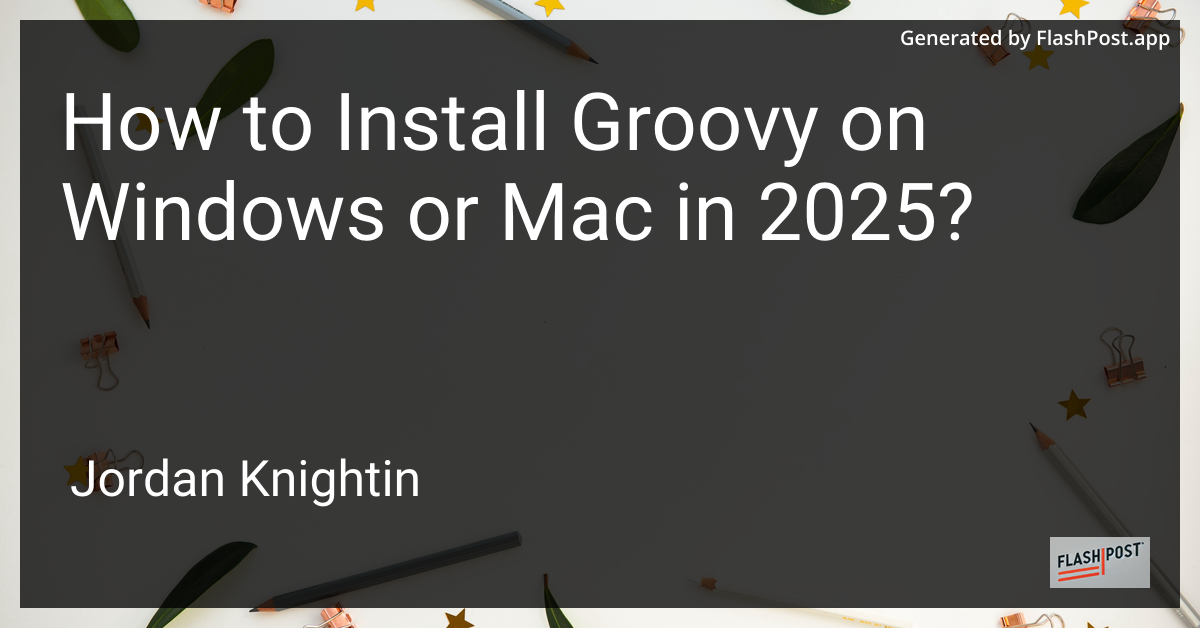
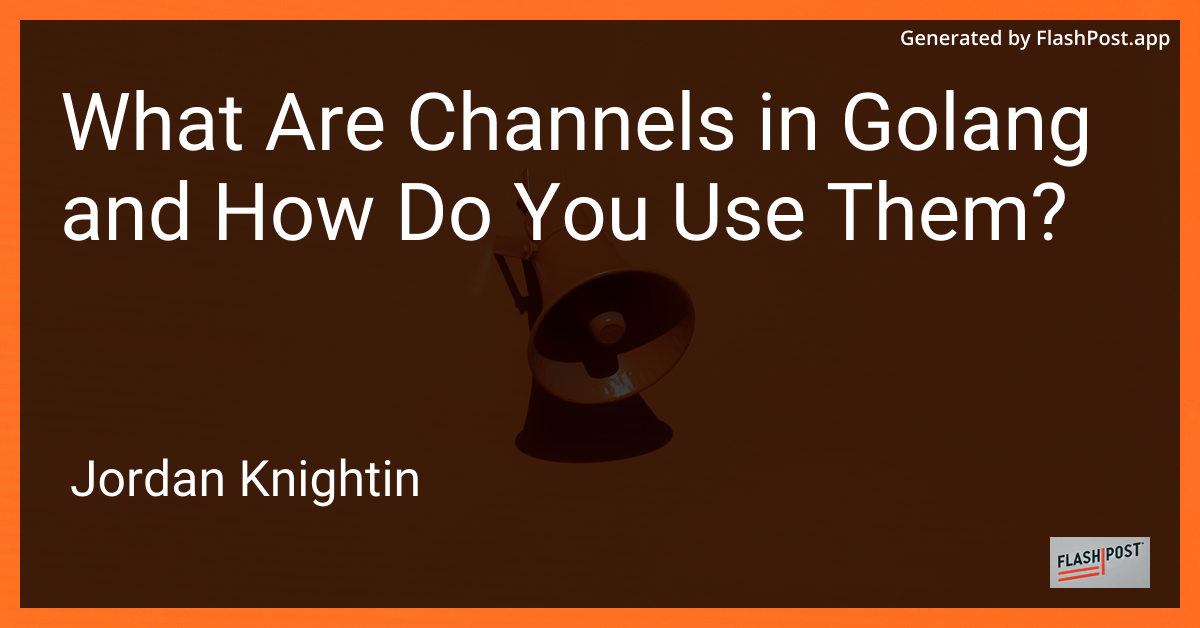
What Are Channels in Golang and How Do You Use Them?
Go, often referred to as Golang, is a statically-typed, compiled language designed for simplicity and efficiency. One of its key features is built-in support for concurrent programming. Channels are a core part of Go’s concurrency model, enabling goroutines to communicate with each other safely. In this article, we will explore what channels are, how they work, and how you can use them effectively in your Go programs.
What are Channels in Go?
Channels in Go are a type-safe conduit for communication between goroutines. They allow goroutines to synchronously exchange data, ensuring that every send is matched by a receive. This mechanism naturally provides a way to synchronize operations across multiple goroutines, avoiding complex locking.
Channels can transmit data of a specified type, making them both flexible and secure in terms of type safety. They support both buffered and unbuffered operations:
- Unbuffered Channels: These block the sending goroutine until another goroutine is ready to receive the sent data, and vice versa. They are excellent for synchronizing events between goroutines.
- Buffered Channels: These allow sending operations to proceed without blocking as long as there is available buffer space, thus enabling more flexibility in communication.
How to Use Channels in Go
Using channels in Go involves several steps: creating channels, sending and receiving data, and closing channels. Here’s a breakdown of these operations:
Creating Channels
You create a channel in Go using the make
function:
ch := make(chan int) // an unbuffered channel of type int
bufferedCh := make(chan int, 10) // a buffered channel with a capacity of 10
Sending and Receiving Data
To send data into a channel, use the <-
operator:
ch <- 42 // Send the integer 42 into the channel
To receive data from a channel, also use the <-
operator:
x := <-ch // Receive data from the channel and assign it to x
Closing Channels
Channels can be closed using the close
function once you are done sending data. This indicates that no more data will be sent through the channel:
close(ch)
Note: It is safe to close a channel from the sender’s side only and typically not required if the channel is just being used for communication without signaling termination.
Practical Example
Here’s a simple example illustrating channels in action:
package main
import (
"fmt"
)
func main() {
messages := make(chan string)
go func() {
messages <- "Hello, Channels!"
}()
msg := <-messages
fmt.Println(msg)
}
In this example, the goroutine sends a string message into the channel, which the main function receives and prints.
Further Resources
Channels are pivotal in writing concurrent programs in Go. Understanding and leveraging them effectively can significantly improve the efficiency and reliability of your applications. To enhance your Go programming knowledge further, consider exploring the following resources:
- Learn more about Golang package installation to manage dependencies effectively.
- Discover tips for Golang web development to build robust web applications.
- Understand best practices for writing unit tests in Golang to ensure the quality and stability of your code.
Conclusion
In Go, channels are an elegant way to handle communication and synchronization between goroutines. Whether you are building simple applications or complex concurrent systems, mastering channels is essential to harnessing the full power of Go. By integrating channels effectively, you can write cleaner, more efficient, and highly concurrent code.