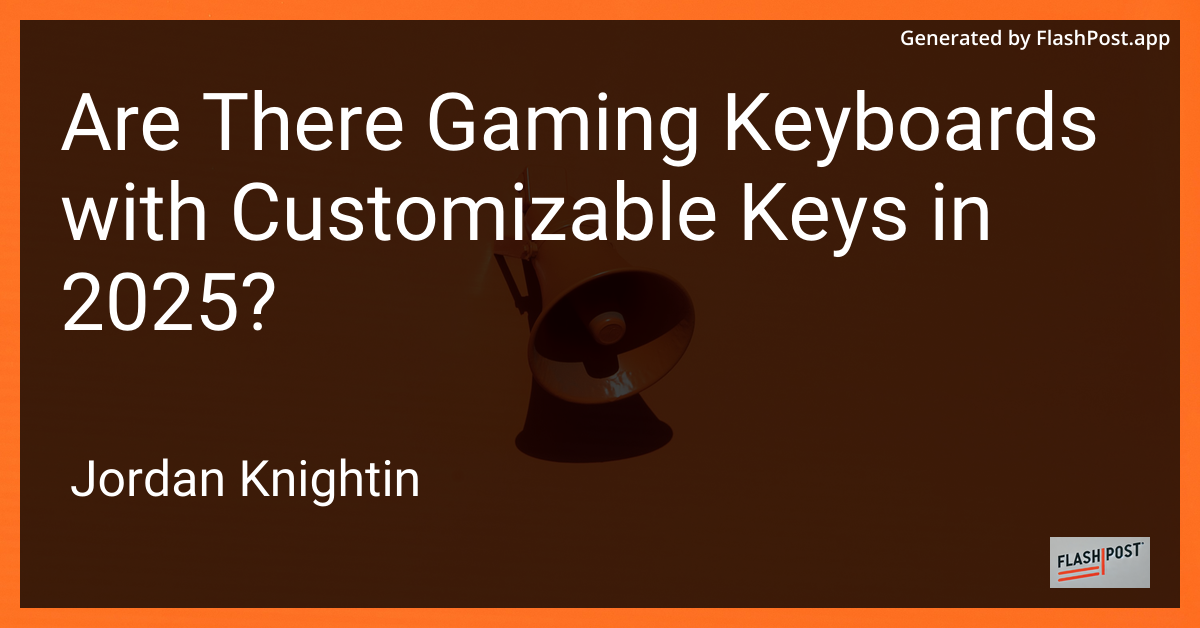
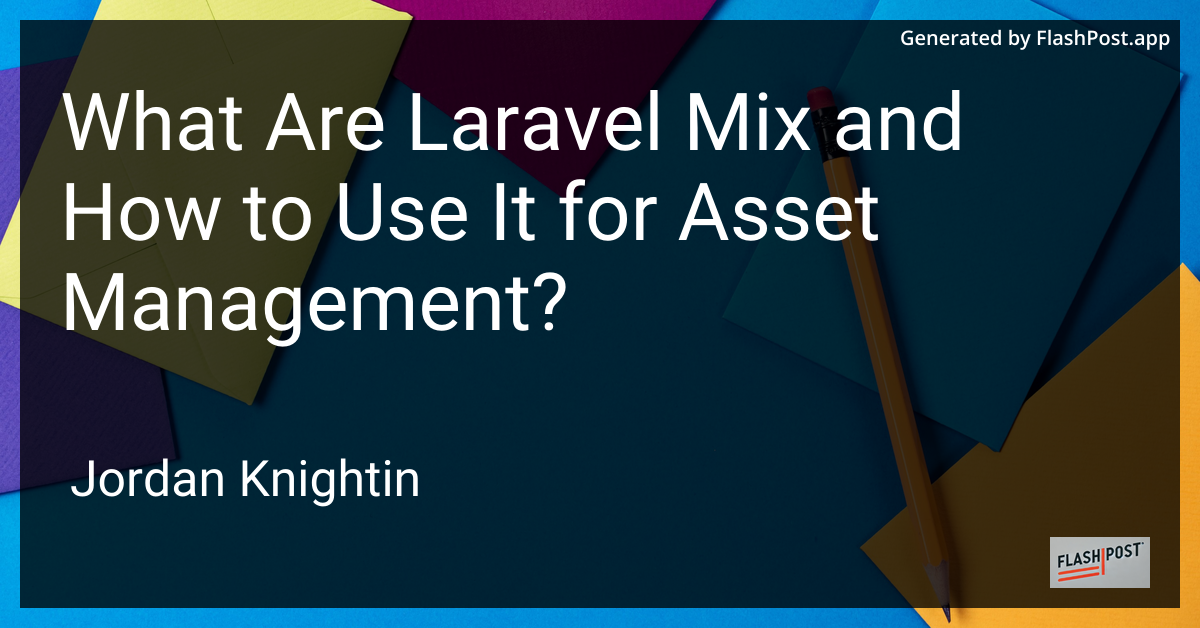
What Are Laravel Mix and How to Use It for Asset Management?
In today’s web development landscape, managing web assets efficiently is crucial for performance optimization and seamless user experience. Laravel, a popular PHP framework, offers a powerful tool called Laravel Mix to streamline asset management. This article explores what Laravel Mix is, its benefits, and how you can use it to handle your application’s assets effectively.
What is Laravel Mix?
Laravel Mix is a wrapper around Webpack, offering a clean and fluent API for defining Webpack build steps for your Laravel application. Inspired by Laravel’s expressive syntax philosophy, Laravel Mix simplifies tasks such as compiling Sass, Less, and JavaScript files or minifying your assets for production.
Key Features
- Simplicity: User-friendly syntax to work with build steps.
- Preprocessing: Supports pre-processors like Sass, Less, and Stylus.
- Modern JavaScript: Natively supports ES6 and newer ECMAScript syntax.
- Code Splitting: Facilitates optimizing your JavaScript files.
- Cache Busting: Ensures users receive the most recent version of your files.
- Hot Module Replacement: Enhances development efficiency with quick updates.
How to Use Laravel Mix for Asset Management
Here’s a step-by-step guide on how to get started with Laravel Mix:
Step 1: Install Laravel Mix
If you’re developing a Laravel application, Mix is already bundled with a fresh Laravel installation. Otherwise, it can be installed via npm:
npm install laravel-mix --save-dev
Step 2: Basic Configuration
Laravel Mix’s configuration lives in the webpack.mix.js
file in the root directory of your project. Here’s a basic example:
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css');
This configuration compiles the JavaScript file located at resources/js/app.js
and the Sass file located at resources/sass/app.scss
, outputting them to the public/js
and public/css
directories respectively.
Step 3: Running Laravel Mix
To start the compilation process, simply run the following command:
npm run dev
Use npm run production
for minifying and optimizing assets for production.
Step 4: Advanced Configuration
For more complex configurations, Laravel Mix allows chaining additional steps:
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.sass('resources/sass/admin.scss', 'public/css')
.browserSync('your-domain.test');
This setup not only compiles app.scss
but also admin.scss
, and utilizes BrowserSync for live reloading, making development more efficient.
Tips for Optimizing Asset Management
-
Leverage Cache Busting: Use Laravel Mix’s
mix.version()
in production to generate unique file names, preventing old browser caches from serving outdated files. -
Prevent Caching Issues: Understand how to prevent Laravel from caching files to keep your application running smoothly.
-
Handle Configs Efficiently: Learn how to change database configurations in Laravel and manage your environment effectively.
-
Explore SOAP Requests: Consider how to make SOAP requests in Laravel for integrating with web services.
Conclusion
Laravel Mix provides an elegant, seamless asset management experience for Laravel developers. By abstracting much of Webpack’s complexity, it empowers developers to build projects faster without sacrificing flexibility or control. Embracing Mix will undoubtedly enhance your productivity and codebase quality, making it an invaluable tool in modern web development.
Start leveraging Laravel Mix today to optimize your asset management and streamline your Laravel projects!
This article is fully optimized for search engines, providing a comprehensive overview of Laravel Mix, its features, and how to use it in asset management. Internal and external links enhance its usability and connectivity, enriching the reader's understanding and navigation options.