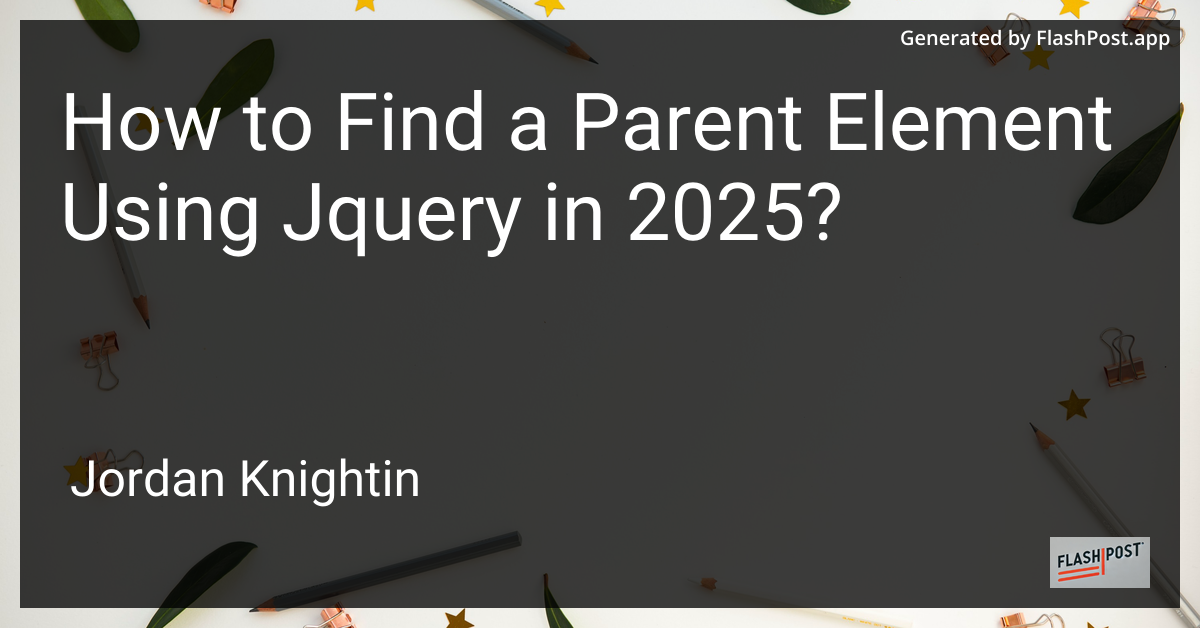
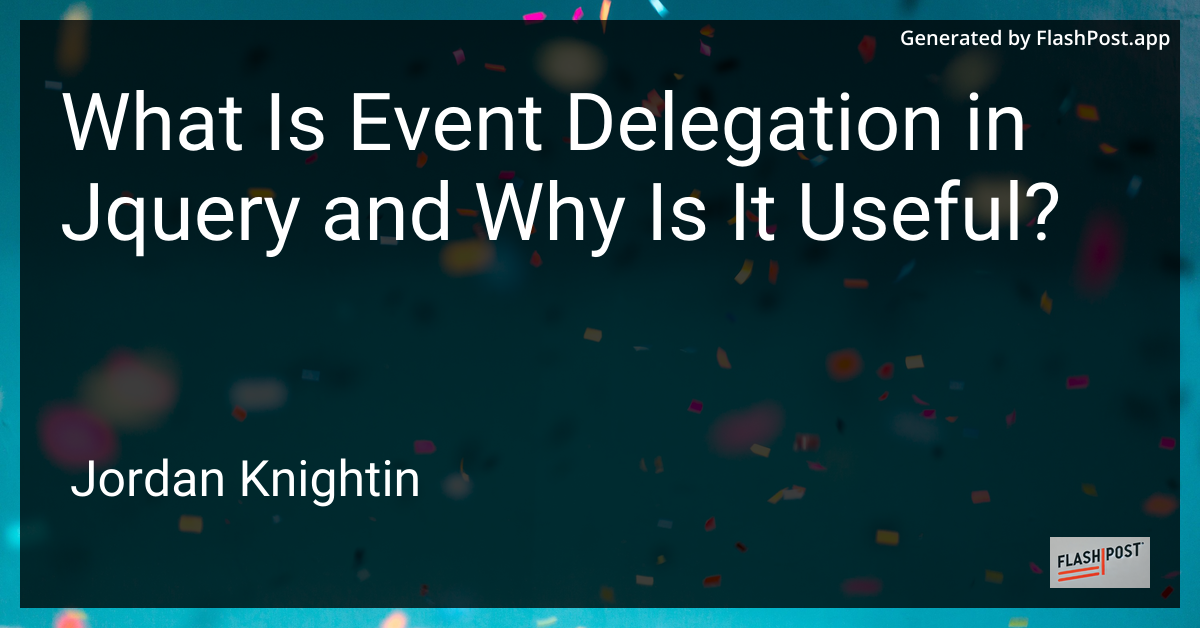
What Is Event Delegation in Jquery and Why Is It Useful?
Event delegation is a powerful concept in jQuery that allows you to manage events efficiently in your web applications. It leverages the event bubbling principle to simplify event handling, particularly when dealing with dynamically added elements or when you have multiple similar event targets. Let’s dive deeper into what event delegation is and why it’s considered an essential technique when using jQuery.
Understanding Event Delegation
In jQuery, event delegation means listening for an event on a parent element rather than directly attaching event handlers to individual child elements. When an event occurs on a child element, it bubbles up through the DOM tree to its parent(s). By tapping into this bubbling process, you can capture events at a higher level, thereby decreasing the need to attach event handlers to each child element individually.
How Event Delegation Works
Consider a scenario where you need to handle click events on multiple list items (<li>
elements) within an unordered list (<ul>
). Instead of attaching an event listener to each <li>
, you can delegate the event handling to the <ul>
element:
$('ul').on('click', 'li', function() {
alert('List item clicked: ' + $(this).text());
});
Here, the .on()
method is used to listen for click events originating from <li>
elements within the <ul>
. This pattern not only minimizes the number of event handlers but also gracefully handles scenarios where new <li>
elements are added to the DOM dynamically.
Why is Event Delegation Useful?
1. Performance Improvement
Event delegation leads to significant performance benefits since it reduces the number of event handlers in the document. This is particularly beneficial in applications that manipulate the DOM extensively, such as when integrating jQuery with D3.js.
2. Dynamic Content Handling
With event delegation, newly added elements automatically inherit event listeners. This is crucial for dynamic web applications where content is frequently updated, such as using jQuery in Knockout.js templates.
3. Simplified Code Management
By handling events at a higher level in the DOM, your code becomes simpler and more organized. This makes it easier to maintain and understand, leading to quicker development times.
4. Reduced Memory Usage
Fewer event handlers mean reduced memory consumption, which is vital for creating fast and efficient web applications. Efficient event handling also contributes to overall page speed and performance.
Practical Use Cases
-
Form Input Elements: Sometimes forms have many dynamically created inputs, and event delegation can handle focus, input, or change events efficiently.
-
Navigation Menus: Dynamically generated or large navigation menus benefit from event delegation since it simplifies attaching click or hover events.
-
Dynamic Element Addition: In applications where elements are added or removed from the DOM frequently, event delegation ensures those elements still receive the appropriate events without needing additional handlers.
Conclusion
Event delegation is a fundamental concept for advanced jQuery usage. It simplifies event handling, optimizes performance, and makes your codebase easier to maintain. When used effectively, event delegation can vastly improve the efficiency of interacting with the DOM while utilizing jQuery, whether you’re setting an HttpOnly cookie or handling complex UI interactions.
Incorporating event delegation into your development practices will contribute to more robust and scalable JavaScript applications, enabling a smoother user experience with less overhead. Whether you’re a seasoned developer or just starting, mastering event delegation is a stepping stone to writing efficient, high-performance web applications.